Programming Logic Explained
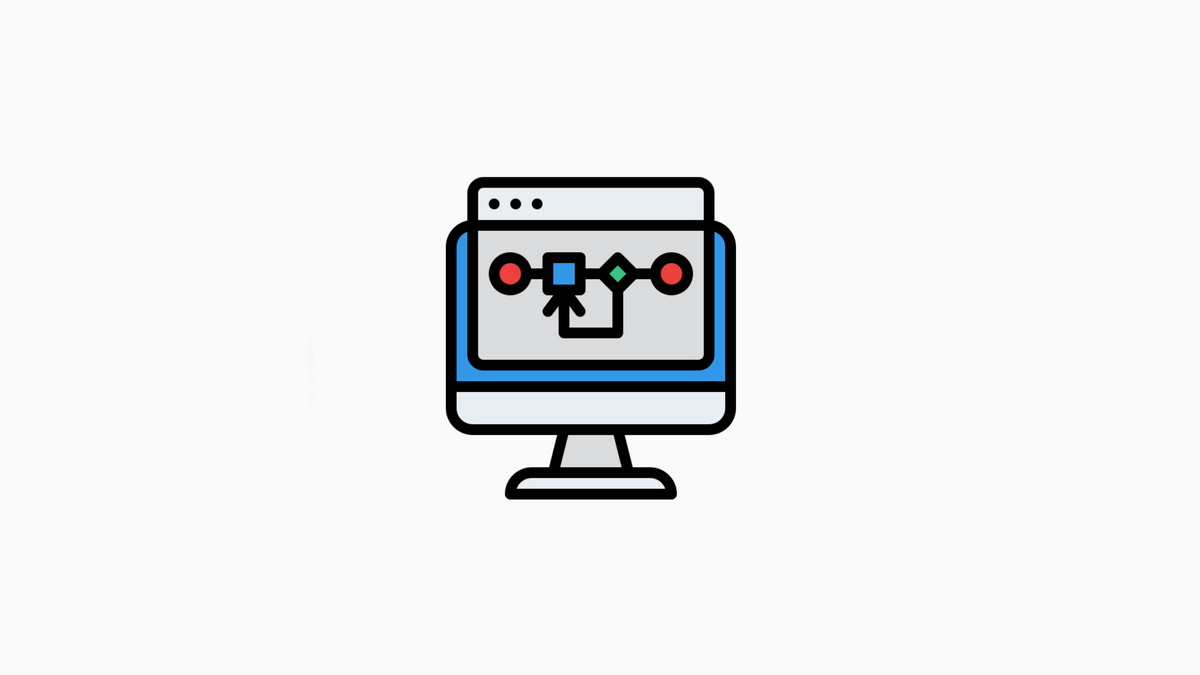
Programming logic is a process used in computer programming to create a step-by-step plan for solving a problem.
It involves breaking the problem into smaller pieces and determining the best way to solve each part. The final issue is then put together from the individual solutions. Logic is also used to design algorithms and step-by-step instructions for carrying out a task.
Flowchart: The basics of a flowchart
Flowcharting is a graphical way of representing the sequence of steps in a program. It can help you see how the different parts of your code fit together, and it can help you find and fix errors in your code.
A flowchart consists of several boxes, called nodes, and arrows between them. The arrows represent the flow of control from one node to the next. The first node is always the starting point, and the last node is always the ending point.
Each node represents a step in the program, and the arrows show how those steps are executed. You can use flowcharts to visualize both sequential and branching code.
Conditionals: If-else statements and switch/case statements
Conditionals are a key part of most programming languages. They allow you to control the flow of your program by executing different codes based on certain conditions. The two most common conditionals are if-else statements and switch/case statements.
An if-else statement looks like this:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
The condition can be anything from a simple variable comparison to a more complex logical expression. If the condition is true, the code in the first block will execute. If the condition is false, the code in the second block will execute.
Loops: For and while loops
Logic is key when programming. In this article, we will discuss the for and while loops. The for loop is used when you know how often you want the code block to run. The while loop is used when you don’t know in advance how many times you want the code block to run. Let’s take a look at some examples:
The for loop is perfect for counting. For example, if I wanted to print out the numbers 1-10, I could use a for loop like this:
for(int i=1;i<=10;i++){ //code block goes here }
This code will execute the code block inside the curly braces ten times, starting with i=1 and incrementing by one each time.
Arrays: One-dimensional arrays
In many programming languages, arrays are one-dimensional. This means that they can only store a list of items linearly. In Python, for example, you create an array by using square brackets, [], and separating the items with commas. So, for example, the following would create an array with five items:
my_array = [1,2,3,4,5]
The first item in the array is at position 0; the second is at position 1, and so on. To access an item in an array, you use the item’s index you want to access. So, for example, to get the value of the third item in my_array, you would use:
my_array[2]
Arrays help store lists of data.
Strings: String manipulation
One of the basic tasks of any programming language is to manipulate strings. This involves taking a string and doing something to it, such as finding its length, extracting a substring, or concatenating two strings together.
Many programming languages provide built-in functions for string manipulation, while others require you to write your code. In either case, it’s important to understand the basic concepts behind string manipulation so that you can write code that is efficient and easy to read and maintain.
Some of the most common string manipulations include:
1) Finding the length of a string: This can be done using the length() function in many programming languages.
2) Extracting a substring from a string: This can be done using various functions provided by different programming languages.
Final Thought
Programming Logic will continue to be an important programming aspect. It is used in programming languages, web development, and many other areas.
While it may change over time, the basics of Programming Logic will always be necessary. Therefore, it is important to stay up-to-date on the latest changes and advancements in this field as a programmer.